Welcome to Backend Development
PHP is a server-side, HTML-embedded scripting language that may be used to create dynamic Web pages. It is available for most operating systems and Web servers, and can access most common databases, including MySQL. PHP may be run as a separate program or compiled as a module for use with a Web server.
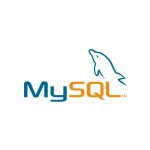
Why Backend Development ?
Open source
Server-side
Relational Database Management
Structured Query Language
Course Content - Backend Development
PHP
- Intro to different kinds of Languages
- Intro to PHP for Web Development
- History & Future Scope of PHP
- Advantage of PHP over JSP
- Installation of Important tools for Working in PHP like WAMP, XAMPP.
Introduction to PHP
- Variables
- Language Construct
- Type Juggling
- Deleting a Variable
- Operators
- Comments
- echo
- if-else
- Loops (for, while)
- switch
Language Basics
- Software development models
- Product Lifecycle Management
- Project Lifecycle
- Waterfall Model
- V-Model
- Iterative – incremental Model
- Testing Levels
- Component Testing
- Integration Testing
- Component Vs System Integration Testing
- Acceptance Testing
- Test types
- Functional testing and Non- functional Testing
- Structural Testing
- Re-Testing and Regression Testing
- Maintenance Testing
- Maintenance Testing Vs New Application Testing
Datatypes
- What is Datatype
- Types of Datatype
- Type Casting
- Garbage Value
Arrays
- What is an Array
- Types of Array
- print_r()
- foreach
- Multi-dimensional Arrays
Functions
- What is a function?
- Types of Function
- return statement
- How to call a function
- Function without parameters
- Function with parameters
- Static Variable
- Difference between Call By Value and Call By Reference
Working with Forms
- What is a Form?
- Important HTML Tags
- Super-Global Variable
- Different ways to carry form data (GET, POST)
- isset()
- isempty()
Regular Expression
- What is Regular Expression?
- Important Symbols used in regular expression with explanation
- Validations
What is a Session?
- Creating a Session
- Use of Session
- Destroying a Session
- Login/Logout
Cookie
- What is a Cookie?
- Difference between Session & Cookie
- Types of Cookie
- Creating a Cookie
- Fetching value of Cookie
- Deleting a Cookie
MySQL
- What is a database?
- What is SQL Injection?
- Different kinds of Datatypes used in MySQL
- Connecting PHP with MySQL
- Creating a Database
- Creating a Table
- insert
- delete
- update
- select
- truncate
- alter
- drop
- grant
- revoke
- commit
- rollback
- rename
- Getting Highest Value from Table
- Getting nth Value from Table
- Uploading Image or File to MySQL
- Retrieve Image or File from MySQL
- Uploading Multiple Files to MySQL
Joins
- What is Join?
- Types of Join
- Practical Implementation
Infycle Technologies
Let Profession Search You